In this section, we'll explore the use of WebSocket in more detail.
Checking for Browser Support
Before you use the WebSocket API, you need to make sure that the browser supports it. This way, you can provide a message, prompting the users of your application to upgrade to a more up-to-date browser. You can use the following code to test for browser support:
Listing 4. Checking for browser support
if (window.WebSocket) {
alert("WebSocket is supported");
} else {
alert("WebSocket is not supported");
}
Listing 4 shows how a call to window.WebSocket returns the WebSocket object if it exists or triggers a failure case if it does not. Figure 5 shows the resulting message in Microsoft Internet Explorer 10, which supports WebSocket.
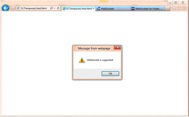
Another way to see if your browser supports WebSocket is to use the browser's developer tools. Figure 5 shows how you can use the WebSocket API from the debug console. You can also test to see if WebSocket is supported there. If it is not, the window.WebSocket command returns "undefined."
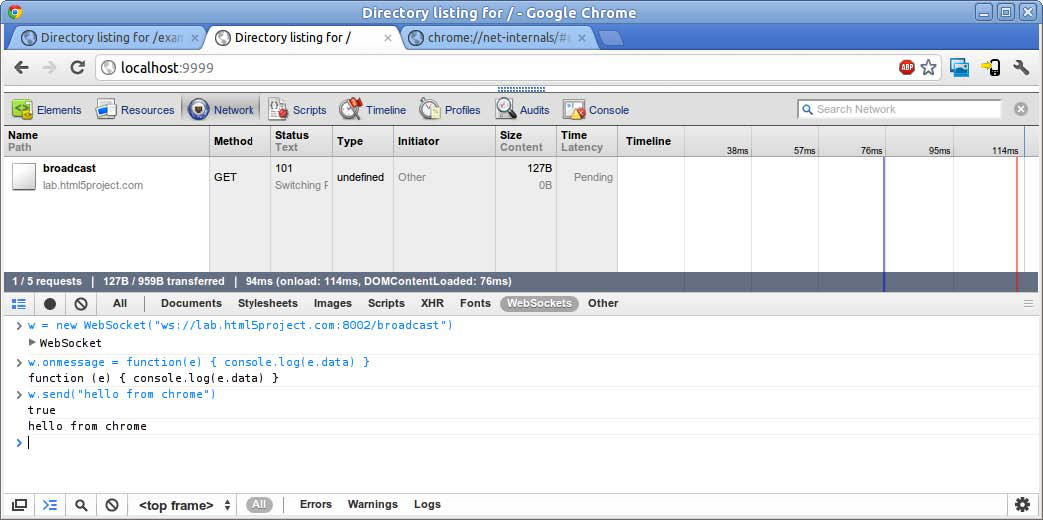
Figure 6. WebSocket connectivity in Chrome Developer Tools' Network panel
In Google Chrome, you can also navigate to chrome://netinternals/# sockets to get fine-grained information about all socket connections as shown in Figure 6.
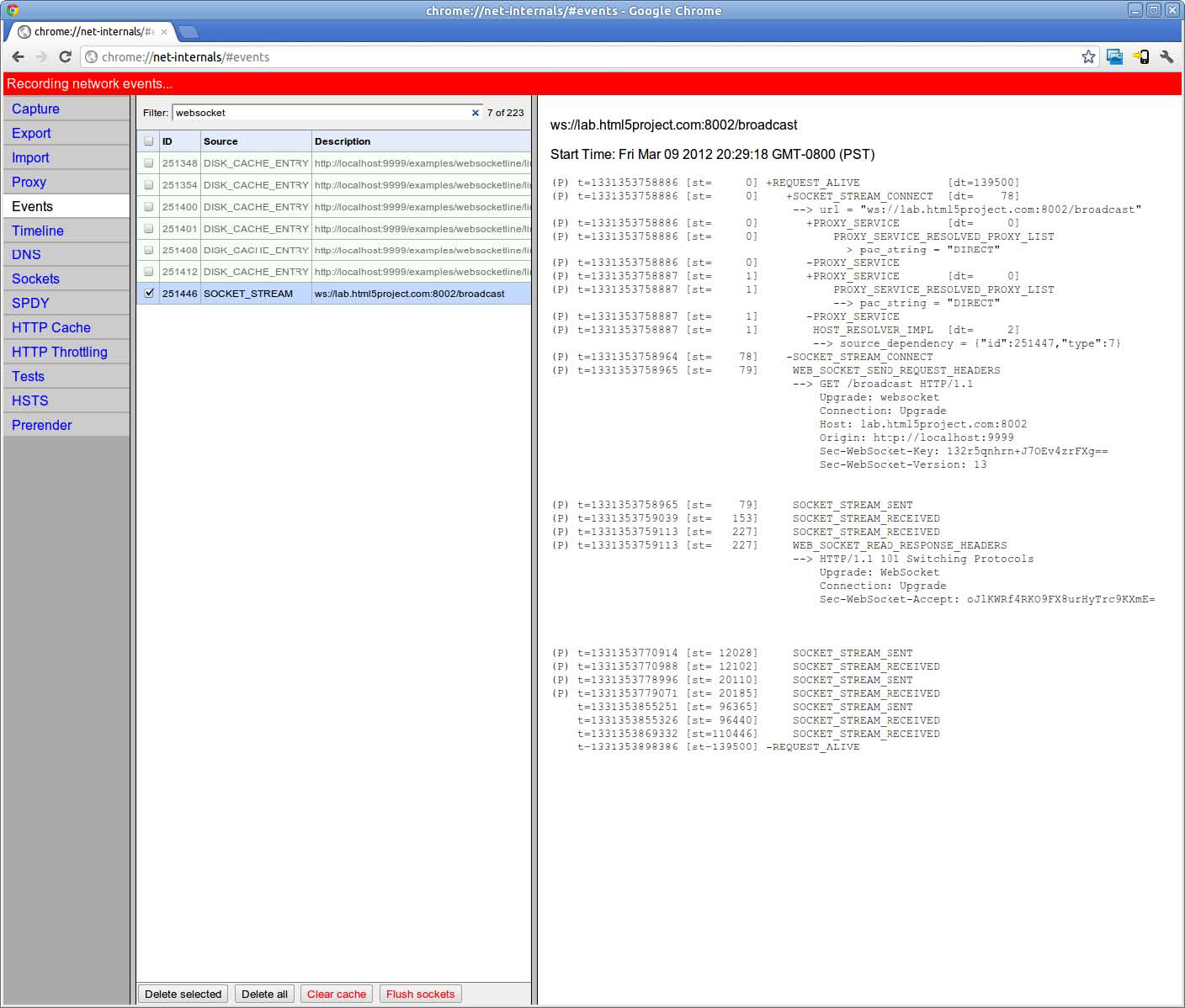
Figure 7. Socket internals page chrome://net-internals/#sockets
Creating a WebSocket object and Connecting to a WebSocket Server
Using the WebSocket interface is quite straightforward. To connect to an endpoint, just create a new WebSocket instance, providing the new object with a URL that represents the endpoint to which you wish to connect. You can use the ws:// and wss:// prefixes to indicate a WebSocket and a WebSocket Secure connection, respectively.
url = "ws://localhost:8080/echo";
w = new WebSocket(url)
When you make a WebSocket connection, you have the option of listing the protocols your application can speak. The second argument to the WebSocket constructor can be a string or array of strings with the names of the subprotocols that your application understands and wishes to use to communicate.
w = new WebSocket(url, protocol);
You can even list several protocols:
w = new WebSocket(url, ["proto1", "proto2"]);
Hypothetically, proto1 and proto2 are well defined protocol names that both the client and server can understand; they may even be registered and standardized. The server will select a prefered protocol from the list. When the socket opens, its protocol property will contain the protocol that the server chooses.
onopen = function(e) {
// determine which protocol the server selected
log(e.target.protocol)
}
Adding Event Listeners
WebSocket programming follows an asynchronous programming model; once you have an open socket, you simply wait for events. You don't have to actively poll the server anymore. You add callback functions to the WebSocket object in order to listen for events.
A WebSocket object dispatches four events: open, message, close, and error. The open event fires when a connection is established, the message event when messages are received, the close event when the WebSocket connection is closed, and the error event when an error occurs. The error event fires in response to unexpected failure. As in most JavaScript APIs, there are corresponding callbacks (onopen, onmessage, onclose, and onerror) that are called when events are dispatched.
w.onopen = function() {
console.log("open");
w.send("Connection open");
}
w.onmessage = function(e) {
console.log(e.data);
}
w.onclose = function(e) {
console.log("closed");
}
w.onerror = function(e) {
console.log("error");
}
Let's take another look at this message handler. The data attribute on the message event is a string if the WebSocket protocol message was encoded as text. For binary messages, data can be either a Blob or an ArrayBuffer, depending on the value of the WebSocket's binaryType property.
w.binaryType = "arraybuffer";
w.onmessage = function(e) {
// data can now be either a string or an ArrayBuffer
console.log(e.data);
}
Sending Messages
While the socket is open (that is, after the onopen listener is called and before the onclose listener is called), you can use the send function to send messages. After sending one or more messages, you can also call close to terminate the connection or you can leave the connection open.
document.getElementById("sendButton").onclick = function() {
w.send(document.getElementById("inputMessage").value);
}
In more advanced uses of WebSocket, you may want to measure how much data is backed up in the outgoing buffer before calling send(). The bufferedAmount attribute represents the number of bytes that have been sent on the WebSocket that have not yet been written onto the network. This could be useful for throttling the rate at which the application sends data.
document.getElementById("sendButton").onclick = function() {
if (w.bufferedAmount < bufferThreshold) {
w.send(document.getElementById("inputMessage").value);
}
}
In addition to strings, WebSocket can also send binary data. This is especially useful when you want to implement binary protocols, such as the standard Internet protocols that are typically layered on top of TCP. The WebSocket API supports the sending of Blob and ArrayBuffer instances as binary data.
var a = new Uint8Array([8,6,7,5,3,0,9]);
w.send(a.buffer);
{{ parent.title || parent.header.title}}
{{ parent.tldr }}
{{ parent.linkDescription }}
{{ parent.urlSource.name }}